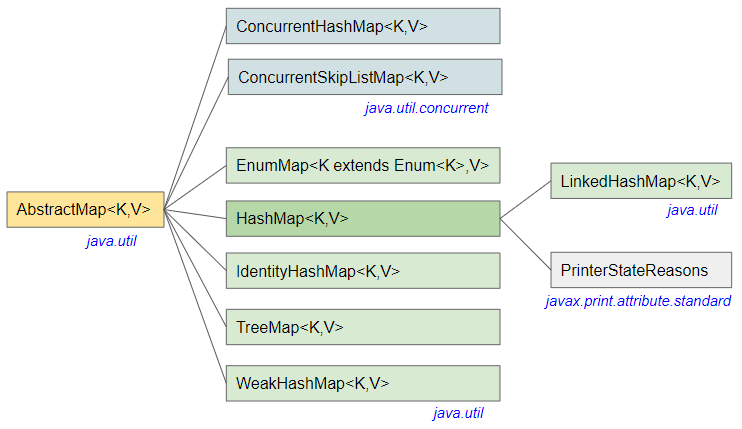
- HashMap contains key and value pairs.
- It can have one null key and multiple null values.
- HashMap does not maintain keys order.
- it works base on hashing technique. (See more explanation of this technique below)
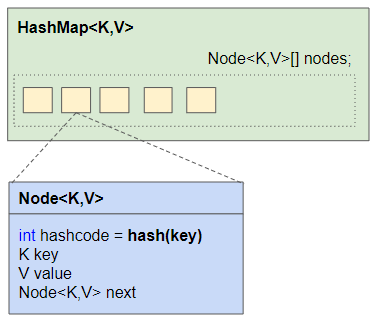
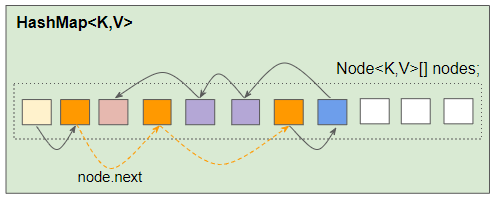
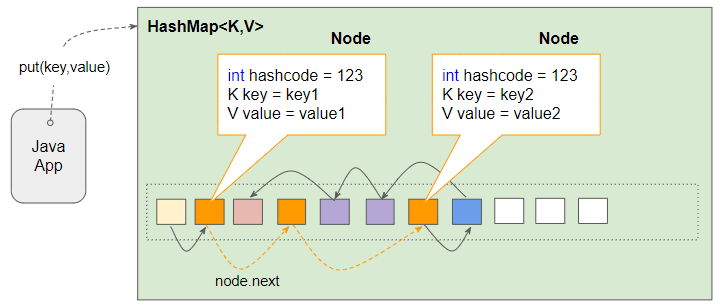
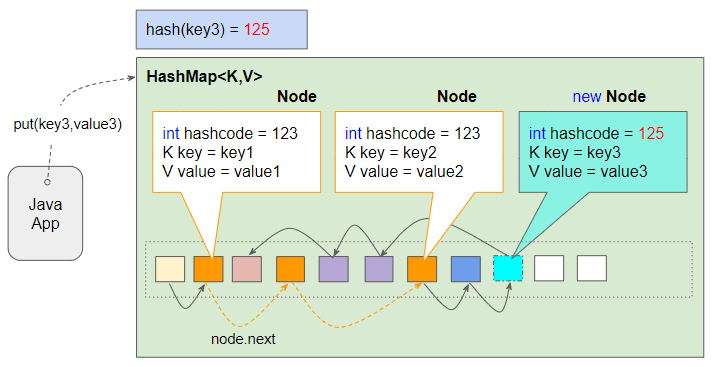
- If the Node corresponding to the key is found, new value will be assigned to Node.value.
- Otherwise, a new Node object is created and assigned to the array (See picture below).
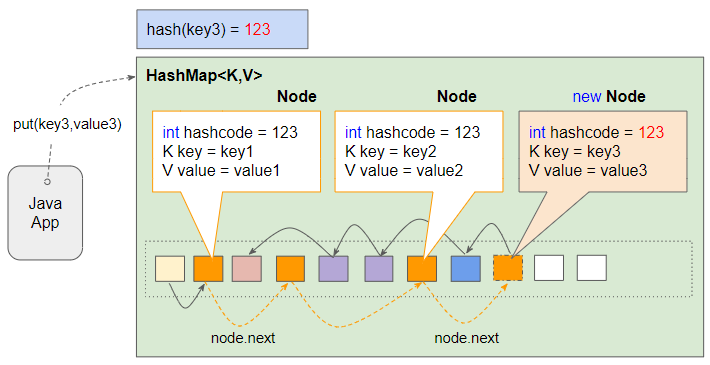
************************************************
✍️AUTHOR: LinkedIn Profile
************************************************
************************************************
✍️AUTHOR: LinkedIn Profile
************************************************
Learn (API-Microservice)Testing+ Selenium UI Automation-SDET with Self Paced Videos prepared by FAANG employee and LIVE Doubt Session
*************************************************
SeleniumWebdriver Automation Testing Interview Questions:
https://automationreinvented.blogspot.com/search/label/SeleniumWebdriver
API Testing Interview Question Set:
https://automationreinvented.blogspot.com/2022/03/top-80-api-testing-interview-questions.html
DevOps Interview Q&A:
https://automationreinvented.blogspot.com/2021/11/top-11-devops-interview-questions-and.html
Kubernetes Interview Question Set
https://automationreinvented.blogspot.com/search/label/Kubernetes
Docker Interview Question Set
https://automationreinvented.blogspot.com/Docker
Linux Interview question Set
https://automationreinvented.blogspot.com/search/label/Linux
Automation Testing/SDET Framework Design
https://automationreinvented.blogspot.com/search/label/FrameworkDesign
Java Related Interview Question Set
https://automationreinvented.blogspot.com/search/label/Java
GIT Interview Question Set:
https://automationreinvented.blogspot.com/2021/09/top-40-git-interview-questions-and.html
Coding Interview Question Set:
https://automationreinvented.blogspot.com/search/label/Coding%20Questions
Mobile Testing Interview Question Set:
https://automationreinvented.blogspot.com/search/label/Mobile%20Testing
Python Interview Question Set for QAE - SDET - SDE:
https://automationreinvented.blogspot.com/search/label/Python
*************************************************
SeleniumWebdriver Automation Testing Interview Questions:
https://automationreinvented.blogspot.com/search/label/SeleniumWebdriver
API Testing Interview Question Set:
https://automationreinvented.blogspot.com/2022/03/top-80-api-testing-interview-questions.html
DevOps Interview Q&A:
https://automationreinvented.blogspot.com/2021/11/top-11-devops-interview-questions-and.html
Kubernetes Interview Question Set
https://automationreinvented.blogspot.com/search/label/Kubernetes
Docker Interview Question Set
https://automationreinvented.blogspot.com/Docker
Linux Interview question Set
https://automationreinvented.blogspot.com/search/label/Linux
Automation Testing/SDET Framework Design
https://automationreinvented.blogspot.com/search/label/FrameworkDesign
Java Related Interview Question Set
https://automationreinvented.blogspot.com/search/label/Java
GIT Interview Question Set:
https://automationreinvented.blogspot.com/2021/09/top-40-git-interview-questions-and.html
Coding Interview Question Set:
https://automationreinvented.blogspot.com/search/label/Coding%20Questions
Mobile Testing Interview Question Set:
https://automationreinvented.blogspot.com/search/label/Mobile%20Testing
Python Interview Question Set for QAE - SDET - SDE:
https://automationreinvented.blogspot.com/search/label/Python
No comments:
Post a Comment